top of page
Recent Posts
Archive
Tags
Swap two words in a byte
#include <iostream> using namespace std; int main() { unsigned int data=0x1234; cout<<"data before swapping :"<<hex<<data<<endl; ...
Fibonacci series
#include <iostream> using namespace std; int main() { // Getting the input of fibonacci series long int n; cout<<"Enter the size of...
Bubble sort (Ascending order) for n number of integers using Dynamic memory allocation.
#include <iostream> using namespace std; void swap(int *a, int *b) { int temp; temp = *a; *a=*b; *b = temp; } void bubblesort(int*...
Difference between diagonal elements in a matrix
#include <math.h> #include <stdio.h> #include <string.h> #include <stdlib.h> #include <assert.h> #include <limits.h> #include...
Maximum number of consecutive 1's in Binary representation of a number
#include <map> #include <set> #include <list> #include <cmath> #include <ctime> #include <deque> #include <queue> #include <stack>...

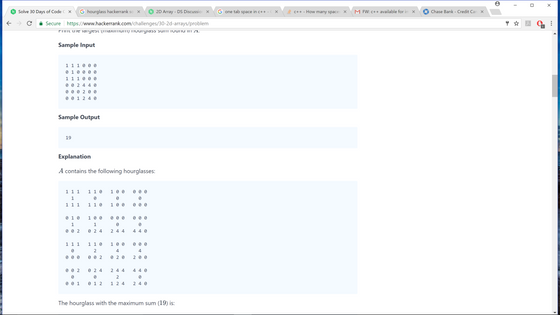
Hourglass Matrix Problem
#include <set> #include <map> #include <list> #include <cmath> #include <ctime> #include <deque> #include <queue> #include <stack>...
Palindrome check using stack and queue
#include <iostream> #include <stack> #include <queue> using namespace std; class Solution { stack<char> s; queue<char> q; // Pushes...
bottom of page